Interested in building your own trivia game? Clone my complete project from GitHub and start making your own trivia app today!
Like I said before, it all started on my porch, about a month before the first big Mardi Gras parades rolled through New Orleans. My sister, my son, and I were casually tossing around trivia — parade schedules, quirky traditions, and odd historical facts. Our casual conversation quickly turned into an exciting idea: What if we created an app to share our Mardi Gras obsession with everyone?
I knew exactly what the app needed:
– An intuitive authentication system to track scores and purchases
– A seamless payment portal
– A robust database of trivia questions and answers
– And, crucially, an attractive and readable user interface
Authentication System
Choosing Firebase for authentication was a no-brainer. With Flutter, integrating Firebase was almost effortless. Here’s a quick rundown:
Firebase Setup:
– Create a Firebase project and register your Flutter app
– Add configuration files (google-services.json for Android, GoogleService-Info.plist for iOS).
1. Flutter Dependencies (`pubspec.yaml`):
yamls
dependencies:
flutter:
sdk: flutter
flutter_login: ^4.0.0
firebase_core: latest_version
firebase_auth: latest_version
2. Initialize Firebase in your App:
void main() async {
WidgetsFlutterBinding.ensureInitialized();
await Firebase.initializeApp();
runApp(MyApp());
}
3. Implement Authentication
Use `flutter_login` for quick setup:
class MyLoginScreen extends StatelessWidget {
final FirebaseAuth _auth = FirebaseAuth.instance;
Future<String?> _login(LoginData data) async {
try {
await _auth.signInWithEmailAndPassword(email: data.name, password: data.password);
return null;
} catch (e) {
return 'Login failed: ${e.toString()}';
}
}
Payment Portal
You can skip this portion if you don’t intend to charge for your game!
Initially, the idea of integrating payments seemed intimidating. I considered Stripe but was bracing myself for a challenge — until I discovered an Invertase extension called “Run Payments with Stripe.” To my surprise, it made the entire setup painless. Within a day, my app was securely processing payments. Users could smoothly unlock new trivia categories or packs, making the transaction experience as seamless as any popular mobile game.
Database for Questions and Answers
Firebase’s Firestore offered a perfect balance between flexibility and scalability, allowing me to store hundreds of questions across various categories. Each trivia question, within a category, was structured clearly:
trivia (collection)
└── category (document)
└── questions (collection)
└── questionID (document)
"question": "Who selected the traditional Mardi Gras colors?",
"options": [
"Rex",
"The Mistick Krewe of Comus",
"King Louis",
"The Cowbellian de Rakin Society"
],
"answer": "Rex",
"level": "Easy"
This setup made managing, updating, and expanding the trivia database incredibly straightforward, even as the content grew richer and more diverse.
Designing for the Parade-goer
The front-end needed special attention. Users would often be playing outside, amidst the buzz of Mardi Gras festivities. I emphasized readability by using high-contrast text on very light backgrounds, ensuring players could clearly see questions even under bright sunlight.
The app awards “stars” for correct answers in the timed solo games, adding a competitive, rewarding element to encourage replayability.

Integrating the Interface: Listing Trivia Categories
After setting up authentication, payment processing, and organizing trivia questions, the next critical step was creating an intuitive way for users to access quizzes. The first step of the trivia experience is a visually appealing list of categories, each expandable to show available quizzes. To make this happen, I created a dedicated widget called TriviaListScreen
.
Structure and Data Flow
To clearly manage the complexity, I separated concerns between my UI (TriviaListScreen
) and my backend (FirestoreService
):
- UI (
TriviaListScreen
): Displays trivia categories, manages user interactions, and reflects user progress and purchases. - Backend (
FirestoreService
): Fetches categories, scores, and question data from Firestore, neatly organized and reusable across the app.
Fetching Data for the Categories List
Here’s how data flows into the interface:
Fetch Categories:
Each trivia category is a Firestore document within the trivia
collection. Categories are ordered by sortOrder
.
Future<List<String>> fetchCategories() async {
QuerySnapshot snapshot = await _db
.collection('trivia')
.orderBy('sortOrder', descending: false)
.get();
return snapshot.docs.map((doc) => doc.id).toList();
}
Levels and Scores:
Each category has multiple levels. I fetch available levels and calculate high scores and question counts for each:
Future<List<int>> fetchAvailableLevels(String category) async {
QuerySnapshot snapshot = await _db
.collection('trivia')
.doc(category)
.collection('questions')
.get();
Set<int> levels = snapshot.docs.map((doc) => doc['level'] as int).toSet();
return levels.toList()..sort();
}
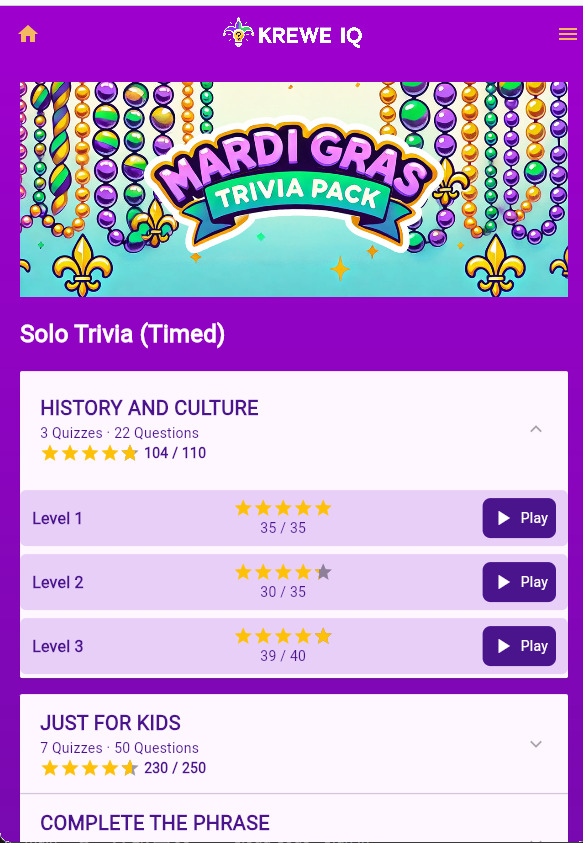
Dynamic User Interface Components
On the frontend, each category is presented using Flutter’s ExpansionPanelList
. This allowed a clean, expandable layout, revealing quiz levels only when a category is tapped.
Real-Time User Progress
The user’s progress, scores, and purchase status dynamically update in real-time. I leveraged Firebase’s snapshot listeners for instant updates:
FirebaseFirestore.instance
.collection('customers')
.doc(user.uid)
.snapshots()
.listen((snapshot) {
if (snapshot.exists && snapshot.data()?['hasPurchased'] == true) {
setState(() {
_hasPurchased = true;
});
}
});
This ensures the app reacts instantly if users purchase premium access.
Smooth Navigation
Using GoRouter
, I set clear navigation pathways. If users clicked on a locked quiz, they seamlessly navigated to the payment screen, enhancing the user experience by removing friction:
onTap: () {
if (!_hasPurchased && category.toLowerCase() != \"sample\") {
GoRouter.of(context).go('/payment');
} else {
String route = widget.isUntimed
? '/untimed/trivia/$category/$level'
: '/trivia/$category/$level';
GoRouter.of(context).go(route);
}
Integrating the Interface: Creating the Quiz
Once a user selects a quiz, the real magic begins. The quiz interface had to be engaging, intuitive, and responsive. Each question needed clear readability, immediate feedback, and a sense of urgency in the timed mode.
Structuring the Questions Screen
I developed a dedicated QuestionsScreen
widget responsible for:
- Fetching questions from Firestore based on selected category and difficulty.
- Managing question navigation and user interactions.
- Displaying real-time scoring and visual feedback through a custom-designed
Scoreboard
and aScoreStats
widget.
Real-Time Timer and Scoring
To amplify engagement, the timed quizzes feature a dynamic countdown timer. The player’s score decreases as the timer progresses, rewarding quicker responses with higher points.
Here’s a simplified view of how the timer logic operates:
void _startCountdownTimer() {
_elapsedTime = 0.0;
_score = 5; // Start with maximum points_timer = Timer.periodic(Duration(milliseconds: 50), (timer) {
setState(() {
_elapsedTime += 0.05;
_score = 5 - (_elapsedTime / (widget.timerDuration / 5)).floor();
if (_score < 0) _score = 0;
})
if (_elapsedTime >= widget.timerDuration) {
_timer?.cancel();
// Automatically proceed or handle timeout
}
});
}
User Feedback and Progress
Selecting a category and level takes users to the QuestionsScreen
, presenting questions fetched from Firestore and shuffled dynamically:
List<String> answers = List.from(question['answers']);
answers.shuffle();
To keep players engaged, each answer triggers a dynamic feedback popup. Correct answers reward the user with stars, clearly displaying their success, while incorrect responses provide immediate, encouraging feedback by showing the correct answer. Here’s a simplified version of this concept:
bool isCorrect = question['correct_answer'] == selectedAnswer;
showDialog(context: context, builder: (context) {
return AlertDialog(
content: Text(isCorrect ? "Correct!" : "Incorrect"),
);
});
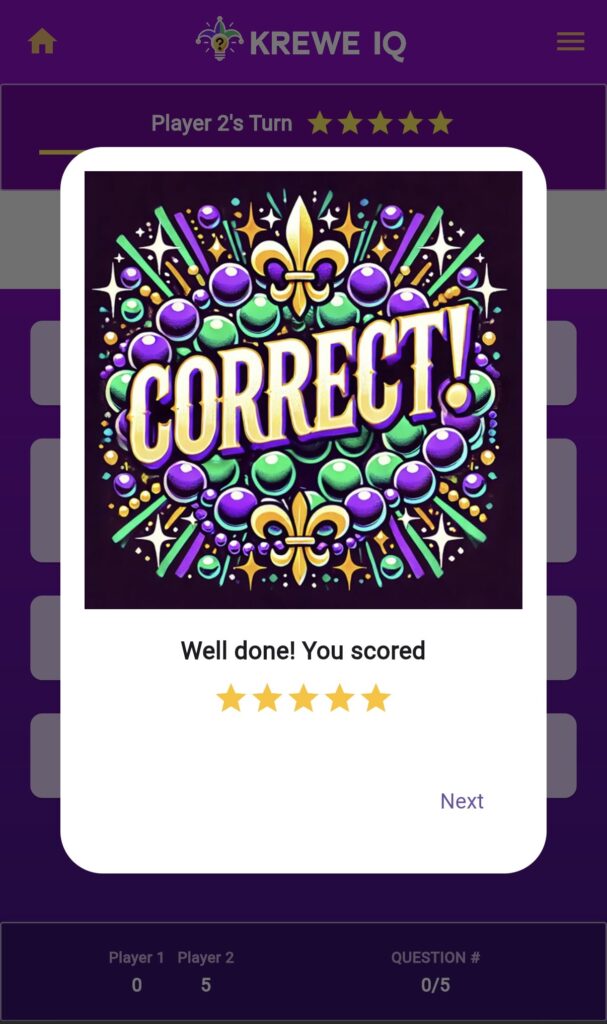
Celebrating Success: Results Screen
After the quiz, users land on the ResultsScreen
, clearly celebrating their achievements and offering options to continue engaging with the app:
Column(
children: [
Text("TOTAL SCORE: \$totalScore"),
ElevatedButton(
onPressed: () => GoRouter.of(context).go('/next-quiz'),
child: Text("Next Quiz"),
),
OutlinedButton(
onPressed: () => GoRouter.of(context).go('/trivia-list'),
child: Text("Back to All Trivia"),
),
],
);
With this results screen, players celebrate achievements clearly and navigate seamlessly to continue the fun.
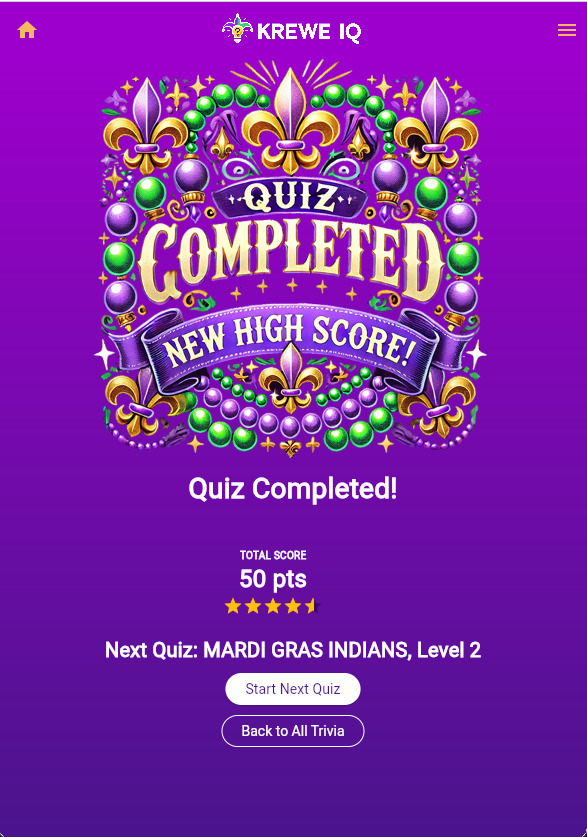
Creating a Mardi Gras trivia app with Flutter, Firebase, and Stripe was a rewarding project that combined my passion for technology and local culture. The seamless integration of user authentication, payment handling, and dynamic content management enabled me to deliver an engaging experience for players. From porch-side idea to parade-ready app, the process was simpler and more enjoyable than I might have imagined.
Interested in building your own trivia game? Clone my complete project from GitHub and start making your own trivia app today!